Master CSS Grid Layout: Centering Content in Cells
To understand how centering works in a grid container, it’s first important to know the grid layout’s structure and scope.
The HTML structure of a grid container consists of three layers:
- The container
- The item
- The content
Each layer operates independently when it comes to applying grid properties.
The scope of a grid container is limited to a parent-child relationship, meaning that a grid container is always the parent and a grid item is always the child. Grid properties are only functional within this relationship.
Any descendants of a grid container beyond the immediate children are not considered part of the grid layout and will not accept grid properties. (However, this can be overridden with the subgrid feature, which allows descendants of grid items to adhere to the lines of the primary container.)
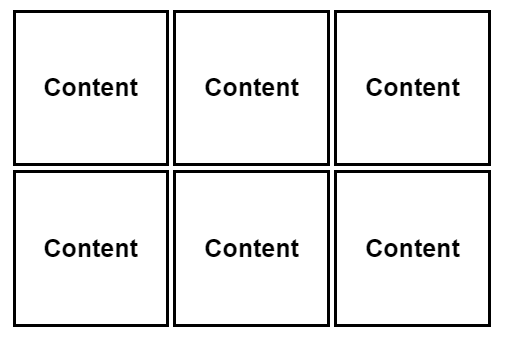
For example, consider a grid container with a simple structure:
HTML
<div class="grid-container">
<div class="grid-item">
<p>Content</p>
</div>
<div class="grid-item">
<p>Content</p>
</div>
<div class="grid-item">
<p>Content</p>
</div>
<div class="grid-item">
<p>Content</p>
</div>
<div class="grid-item">
<p>Content</p>
</div>
<div class="grid-item">
<p>Content</p>
</div>
</div>
In this code snippet,
- The
grid-container
is the parent, thegrid-item
is the child, and thep
tag represents the content. - The grid properties applied to the
grid-container
will affect the layout of thegrid-item
, but not thep
tag. - This is because the
p
tag is a descendant, not a direct child, of thegrid-container
.
CSS
.grid-container{
display: inline-grid;
grid-template-rows: 100px 100px 100px;
grid-template-columns: 100px 100px 100px;
grid-gap: 3px;
}
.grid-item
{
display: flex;
justify-content: center;
align-items: center;
border: 2px solid black;
font-size: 3em;
}
Let’s break this code to understand
.grid-container
: This class represents a container that uses an inline grid display. It’s like a grid paper where you can place items.
grid-template-rows
and grid-template-columns
: These properties define the rows and columns of the grid. In our example, there are three rows and three columns, each with a size of 100 pixels.
grid-gap
: This property sets the gap (spacing) between grid items. In your case, it’s 3 pixels.
.grid-item
: This class represents an item within the grid. It’s a flex container (using display: flex
) that centers its content both horizontally (justify-content: center
) and vertically (align-items: center
). The border is 2 pixels wide, and the font size is 3em.
You can use this code, as a reference to build a grid with evenly spaced rows and columns, and each item inside the grid will be centered both horizontally and vertically. 😊
See the Pen Centering in CSS Grid by Jaspreet (@jasjain) on CodePen.
About the author
Jaspreet Kaur
Jaspreet is a UI/UX designer with expertise in front-end development for web and mobile. She is passionate about designing solutions through collaboration, iteration, and following the best technology development practices.